To Select a dropdown value with selenium webdriver you have to create a Select Element.
First import a package
import org.openqa.selenium.support.ui.Select;
Setting a WebElement dropdown to the select dropdown menu using Xpath
WebElement dropdown=driver.findElement(By.xpath("/html/body/div/div/div[2]/div
/div[2]/div[1]/div[3]/div[1]/div[1]/div/select"));
Creating a Select Element and passing WebElement as a parameter
Select sel=new Select(dropdown);
Now there are 3 ways in which we can select option from select dropdown menu
1. SelectByVisibileText - sel.selectByVisibileText("hey");
2. SelectByIndex - sel.selectByIndex(1); //1 will select 1st option
3. SelectByValue - sel.selectByValue("Faculty");
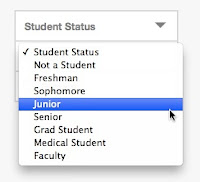
import org.openqa.selenium.support.ui.Select;
public class select1{
public static void main(String args[]) throws IOException
{
System.setProperty("webdriver.chrome.driver","C:/Users/Desktop/chromedriver.exe");
WebDriver driver=new ChromeDriver();
driver.get("any website which have select dropdown");
WebElement a=driver.findElement(By.xpath("/html/body/div/div/div[2]/div/div[2]
/div[1]/div[3]/div[1]/div[1]/div/select"));
Select sel=new Select(a);
sel.selectByIndex(1);
System.out.println(sel.getText());
}
}
First import a package
import org.openqa.selenium.support.ui.Select;
Setting a WebElement dropdown to the select dropdown menu using Xpath
WebElement dropdown=driver.findElement(By.xpath("/html/body/div/div/div[2]/div
/div[2]/div[1]/div[3]/div[1]/div[1]/div/select"));
Creating a Select Element and passing WebElement as a parameter
Select sel=new Select(dropdown);
Now there are 3 ways in which we can select option from select dropdown menu
1. SelectByVisibileText - sel.selectByVisibileText("hey");
2. SelectByIndex - sel.selectByIndex(1); //1 will select 1st option
3. SelectByValue - sel.selectByValue("Faculty");
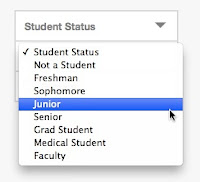
Script
public class select1{
public static void main(String args[]) throws IOException
{
System.setProperty("webdriver.chrome.driver","C:/Users/Desktop/chromedriver.exe");
WebDriver driver=new ChromeDriver();
driver.get("any website which have select dropdown");
WebElement a=driver.findElement(By.xpath("/html/body/div/div/div[2]/div/div[2]
/div[1]/div[3]/div[1]/div[1]/div/select"));
Select sel=new Select(a);
sel.selectByIndex(1);
System.out.println(sel.getText());
}
}
0 comments: